Data Structures and Algorithms (DSA)
Enroll Now and Become a Certified with Us
★★★★★ 5/5
- 4.721 students
- Last updated 25/7/2023
Descriptions
Data Structures and Algorithms (DSA) are fundamental to computer science and software development, enabling efficient data organization, management, and processing. Data structures like arrays, linked lists, stacks, queues, trees, and graphs help perform operations such as searching, sorting, and manipulation. Algorithms provide step-by-step procedures for solving problems, from sorting data to finding the shortest paths. Mastering DSA is vital for optimizing code, enhancing performance, and building scalable applications. It equips developers to tackle complex computational challenges effectively and excel in technical interviews and software development.
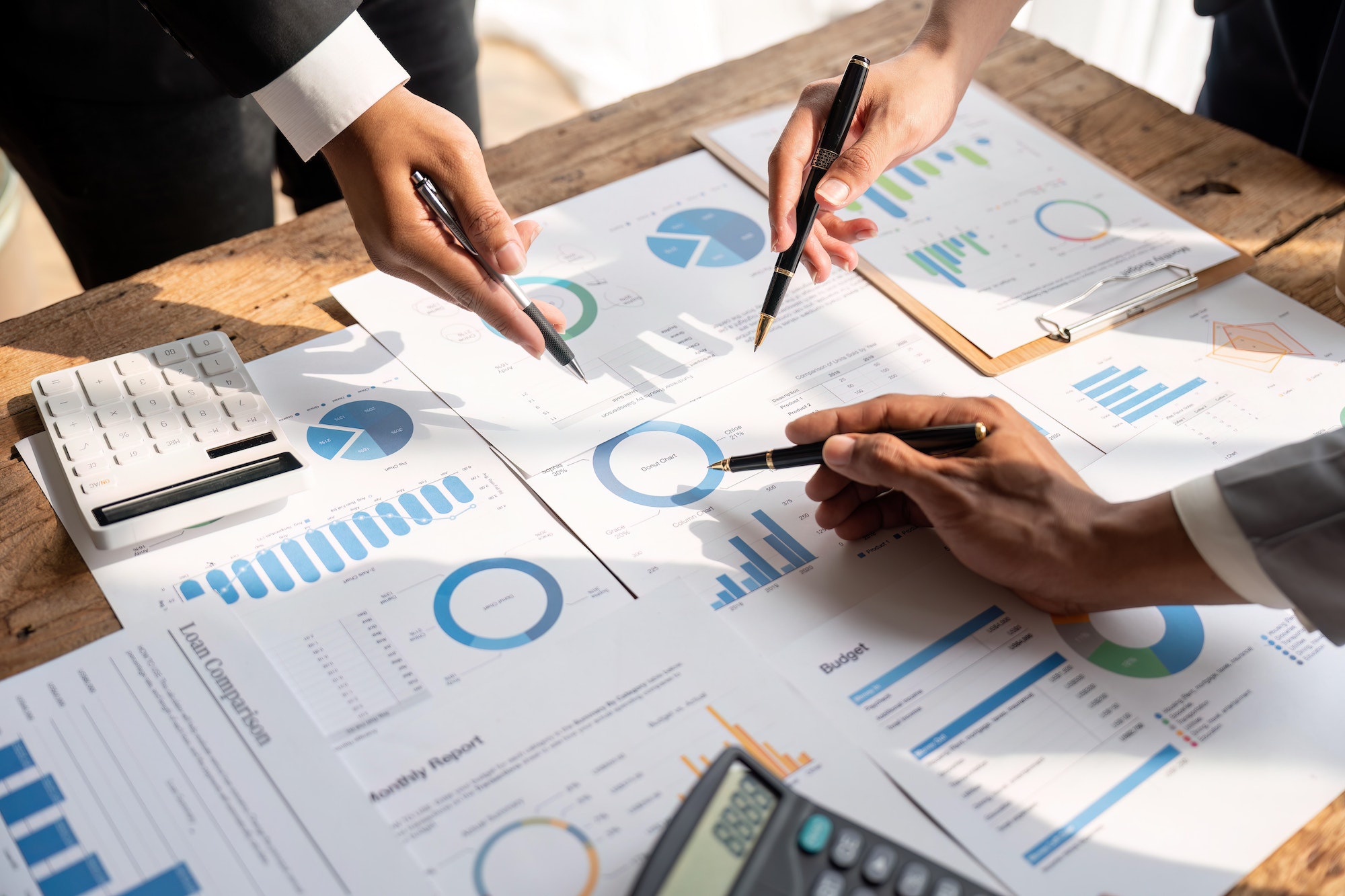
Key Points
- Comprehensive Coverage of DSA Concepts
- Hands-On Practice with Data Structures
- Mastering Algorithmic Techniques
- Real-World Problem Solving
- Technical Interview Preparation
- Industry-Experienced Instructors
Course Lessons
Data Structures and Algorithms (DSA) are fundamental components of computer science and software development. They provide the necessary tools to organize, manage, and store data efficiently, enabling effective data access and modification. A solid grasp of DSA is essential for optimizing code performance, building scalable applications, and excelling in technical interviews.
This module introduces the core concepts of data structures and algorithms, including their definitions, classifications, and the Abstract Data Type (ADT) model. Students will learn about algorithm representation, complexity analysis, and memory management techniques such as dynamic allocation using malloc, realloc, and calloc.
Focusing on stack data structures, this section covers their implementation and practical uses. Topics include infix, prefix, and postfix expression conversions, as well as the evaluation of these expressions using stack operations.
Students will explore various types of linked lists, including singly, doubly, and circular linked lists, along with their operations. The module also delves into queue implementations, covering standard queues, circular queues, and priority queues, highlighting their applications in real-world scenarios.
A comprehensive look at fundamental searching and sorting techniques is provided in this section. Topics include binary search, selection sort, insertion sort, merge sort, quicksort, and quickselect algorithms, emphasizing their implementation details and performance analysis
Focusing on efficient data retrieval, this section covers hashing techniques, including chaining methods and the creation of perfect hashing functions. Additionally, students will explore string manipulation algorithms and pattern searching methods, such as the Rabin-Karp algorithm, to handle complex string operations effectively.
This module introduces graph and tree data structures, exploring their properties and traversal methods. Students will learn about heaps and heap sort, priority queues, binary trees, n-ary trees, binary search trees, and trie trees. The module also covers advanced topics such as Kruskal’s Minimum Spanning Tree algorithm, Dijkstra's shortest path algorithm, disjoint sets, Floyd-Warshall's algorithm, breadth-first search (BFS), depth-first search (DFS), AVL trees, B-trees, and threaded trees.
Instructor
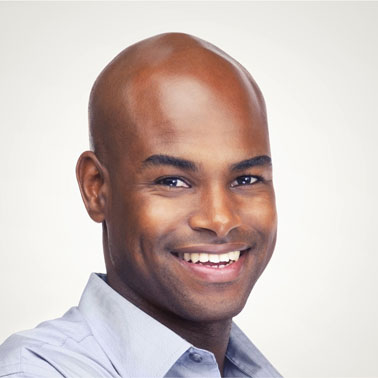
Joshua Hamilton
Data Science Expert
This course includes:
- 62 hours on-demand video
- Full lifetime access
- Access on mobile and TV
- Free Webinar
- Certificate of completion